Clang-Repl¶
Clang-Repl is an interactive C++ interpreter that allows for incremental compilation. It supports interactive programming for C++ in a read-evaluate-print-loop (REPL) style. It uses Clang as a library to compile the high level programming language into LLVM IR. Then the LLVM IR is executed by the LLVM just-in-time (JIT) infrastructure.
Clang-Repl is suitable for exploratory programming and in places where time to insight is important. Clang-Repl is a project inspired by the work in Cling, a LLVM-based C/C++ interpreter developed by the field of high energy physics and used by the scientific data analysis framework ROOT. Clang-Repl allows to move parts of Cling upstream, making them useful and available to a broader audience.
Clang-Repl Usage¶
clang-repl> #include <iostream>
clang-repl> int f() { std::cout << "Hello Interpreted World!\n"; return 0; }
clang-repl> auto r = f();
// Prints Hello Interpreted World!
Note that the implementation is not complete and highly experimental. We do not yet support statements on the global scope, for example.
Clang-Repl Basic Data Flow¶
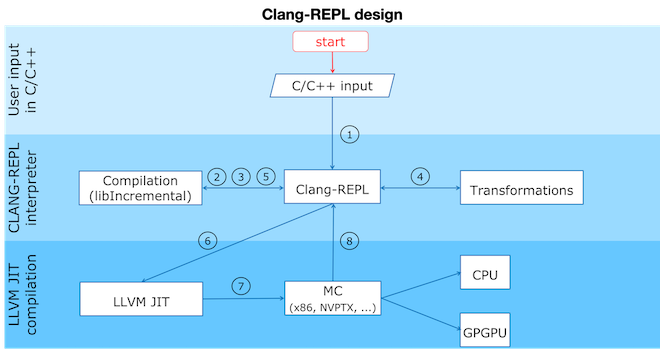
Clang-Repl data flow can be divided into roughly 8 phases:
Clang-Repl controls the input infrastructure by an interactive prompt or by an interface allowing the incremental processing of input.
Then it sends the input to the underlying incremental facilities in Clang infrastructure.
Clang compiles the input into an AST representation.
When required the AST can be further transformed in order to attach specific behavior.
The AST representation is then lowered to LLVM IR.
The LLVM IR is the input format for LLVM’s JIT compilation infrastructure. The tool will instruct the JIT to run specified functions, translating them into machine code targeting the underlying device architecture (eg. Intel x86 or NVPTX).
The LLVM JIT lowers the LLVM IR to machine code.
The machine code is then executed.
Just like Clang, Clang-Repl can be integrated in existing applications as a library (via using the clangInterpreter library). This turning your C++ compiler into a service which incrementally can consume and execute code. The Compiler as A Service (CaaS) concept helps supporting move advanced use cases such as template instantiations on demand and automatic language interoperability. It also helps static languages such as C/C++ become apt for data science.